一、命令行使用
redis大概有200多命令,这里只是入门级别,列举了一些非常常见的内容,如果这些会了就可以开启redis进一步学习了。
1、登录数据库
我们需要知道ip地址、端口号、密码(如果有)。
登录命令:
有密码:
1
| redis-cli -h 127.0.0.1 -p 6379 -a "bunny"
|
无密码:
1
| redis-cli -h 127.0.0.1 -p 6379
|
本机登录可以直接:
如果要正确显示中文,需要加上参数 –raw
2、字符串数据操作
存储,如果存在就更新
1 2
| set name bunny set age 11
|
批量存储
1
| mset name kk password 123456
|
增加,不修改,如果key存在则失败。
字符串内容后追加“2020”
数字内容增加1
数字内容减少1
读取
删除
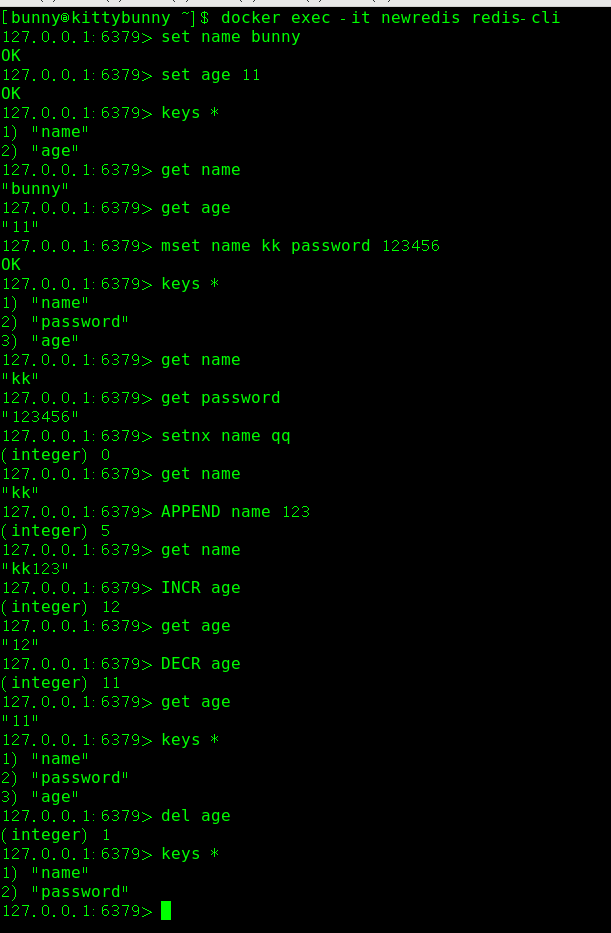
3、列表数据操作-list
从左侧添加数据(可以多个,从前面的值开始添加,相当于多个命令)
从左侧删除数据并返回该数据
从右侧添加数据(可以多个)
从右侧删除数据并返回该数据
根据索引修改值(0开始)
根据索引获取值(0开始)
获取列表长度
显示范围值(闭区间)
4、集合数据操作-set
向集合添加一个或多个成员
1 2
| sadd myset 1 2 sadd myset tom
|
返回集合中的所有成员
移除并返回集合中的一个随机元素
判断 member 元素是否是集合 key 的成员
5、对象存储-hash
student(id:1,no:bunny001,name:kitty,sex:男)
存储上面的对象:
1 2
| hset student id 1 hmset student no bunny001 name kitty sex 男
|
获取内容:
1 2 3
| hget student no //获取单一值 hmget student id sex //获取多个值 hgetall student //获取所有值,结果必然偶数行,key val交替。
|
显示所有的属性
显示所有的值
删除属性
6、其他
删除数据
清空当前数据库
清空所有数据库
获取数据类型
设置过期时间
10秒过期,不存在值才能成功
二、jedis使用
svn://www.kittybunny.cn/kitty/2、code/redis 用户名密码: reader reader
1、添加2个jar
commons-pool2-2.4.2.jar
jedis-2.9.0.jar
2、测试连接
1 2 3 4 5
| Jedis jedis = new Jedis("127.0.0.1", 16379); // jedis.auth(""); //密码 jedis.set("kitty", "bunny"); System.out.println(jedis.get("kitty")); jedis.close();
|
3、操作string
1 2 3 4 5
| Jedis jedis = new Jedis("127.0.0.1", 16379); // jedis.auth(""); //密码 jedis.set("string01", "mystring"); System.out.println(jedis.get("string01")); jedis.close();
|
4、操作list
1 2 3 4 5 6 7 8
| Jedis jedis = new Jedis("127.0.0.1", 16379); // jedis.auth(""); //密码 jedis.lpush("javalist", "s1"); jedis.lpush("javalist", "s2", "s3"); System.out.println(jedis.lrange("javalist", 0, -1)); System.out.println(jedis.lpop("javalist")); System.out.println(jedis.lrange("javalist", 0, -1)); jedis.close();
|
5、操作set
1 2 3 4 5
| Jedis jedis = new Jedis("127.0.0.1", 16379); // jedis.auth(""); //密码 jedis.sadd("javaset", "s1", "s2"); System.out.println(jedis.smembers("javaset")); jedis.close();
|
6、操作hash
1 2 3 4 5 6 7 8 9 10
| Jedis jedis = new Jedis("127.0.0.1", 16379); // jedis.auth(""); //密码 jedis.hset("user", "id", "oo1"); HashMap<String, String> hash = new HashMap<>(); hash.put("name", "名字"); hash.put("password", "pwd"); hash.put("sex", "m"); jedis.hmset("user", hash); System.out.println(jedis.hgetAll("user")); jedis.close();
|
7、连接池操作
1 2 3 4 5 6 7 8
| JedisPoolConfig pool = new JedisPoolConfig(); pool.setMaxTotal(5);//最大连接 pool.setMaxIdle(1);//最大空闲 JedisPool jp = new JedisPool(pool, "127.0.0.1", 16379); Jedis jedis = jp.getResource(); // jedis.auth(""); //密码 System.out.println(jedis.ping()); jedis.close();
|
8、当作缓存使用
1 2 3 4 5 6 7 8 9 10 11 12
| Jedis jedis = new Jedis("127.0.0.1", 16379); // jedis.auth(""); //密码 String key = "sessionid"; if (jedis.exists(key)) { System.out.println(jedis.get(key)); } else { // 查询数据库,oracle或者mysql String content = "数据库中内容"; jedis.set(key, content); System.out.println("数据库中查询到:" + content); } jedis.close();
|